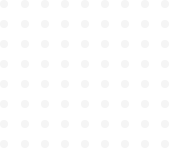
Personal Portfolio Landing Page
Advance CSS & Responsive Design
CSS Animation
Because the skillset is the most plain section, we’ll add animation to that. We’ll add a glow to the skill containers.
- Animations are used to create dynamic effects on elements, by changing their style gradually over a defined time
- Animations in CSS are defined using
@keyframes
rule specifying the style change at different stages.
@keyframes glow { 0% { box-shadow: 0 0 5px #7a7878; } 100% { box-shadow: 0 0 15px #7a7878, 0 0 25px #7a7878, 0 0 35px #7a7878; } }
- Now that animation has been defined, it has to be applied to the respective elements
.skillset h4:hover { animation: glow 1s infinite alternate; } /*animation: name duration iteration-count direction;*/
CSS Transition
The CSS transition
property helps make things change smoothly on a webpage, When you hover over a button, it can change color without looking abrupt, so it almost gives an animated effect to that change.
transition: property duration timing-function delay;
Let’s try this on the navigation links. And the buttons.
nav a {
text-decoration: none;
color: #fb8460;
transition: color 0.3s ease-out;
}
We just need to add this transition property to the generic button element, it’ll be applied to all three types of buttons.
button {
padding: 0.8rem 1.5rem;
width: max-content;
border-radius: 2.5rem;
border: none;
background-color: var(--primary-color);
color: white;
margin-right: 1rem;
transition: all 0.2s ease-in;
}
Responsive Web Design
We have created the website so far with a desktop-first approach. This means, that it isn't going to look as good on the smaller screens.
Responsive design means writing CSS such that the website design is consistent across all media screens.
Viewport Meta Tag
The viewport meta tag is a special HTML meta tag used to control how a webpage is displayed on different devices, especially on mobile devices. It helps ensure that your website looks and functions well regardless of screen size and orientation.
<meta name="viewport" content="width=device-width, initial-scale=1.0">
Here’s the breakdown of the Viewport meta tag,
- Meta Tag in HTML:
- The viewport meta tag is placed within the
<head>
section of your HTML document.
- The viewport meta tag is placed within the
- Viewport Configuration:
- The
content
attribute of the viewport meta tag is used to configure how the viewport behaves.
- The
- Width and Initial Scale:
width=device-width
sets the width of the viewport to the device's width.initial-scale=1.0
ensures that the website is initially displayed at its original scale, without any zooming.
Media Queries
Adaptive Styling: Media queries let you define different stylings for different screen sizes.
Here are the key parts:
- Device Conditions: Media queries check things like screen width, height, and orientation (portrait or landscape) to figure out what kind of device is being used.
- CSS Rules: Once the query figures out the device, it applies specific sets of rules (styles) to make the website look good on that device.
Syntax: Media queries use the @media
rule in CSS, followed by conditions in parentheses and curly braces. Inside the curly braces, you define the styles that apply when the conditions are met.
Let’s start with the global design.
@media (max-width: 600px) {
body {
font-size: 13px;
}
}
Note:
- In a media query, the "screen" keyword specifies the type of media or device for which the styles inside the query should apply.
- Inside the query, you can use queries like
max-width
,min-width
,max-height
,min-height
,orientation: landscape.
- The absolute value in media query is called the breakpoint
Although we’ll figure out everything on the way, I have made the notes for what we might have to change.
Adding Responsiveness
Navbar
The changes needed for the mobile, tab layout
- nav element’s flex-direction from row to column
- alignment of the nav content on the center horizontally, which is on cross axis, so use align-items
- Reduce the size of nav elements and the space they take up, to align them on the same line
Hero section
Again, we can stack the two divisions of the header section, to make it look more presentable.
- Change hero-element flex-direction to column
- align-items on end
- add verticle gap
- Increase the width of header divisions to fit the mobile screen
About
- change direction to the column, or column reverse whatever suits you
- increase text division to 90% from 60% width of container width
Projects
- Change flex-direction to the column, no change for tabs
- Reduce padding and gap for mobile
- Increase text img division sizes inside project card to 100%
Contact
- Increase block size to 100%
- increase the width of input elements