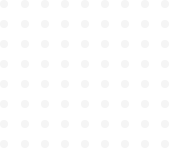
Personal Portfolio Landing Page
CSS Fundamentals and Styling
Introduction to CSS
- Cascading Style Sheets is a stylesheet language
- It gives a set of instructions for how an element is going to be presented on the web page
- Focuses on the appearance, layout, and aesthetics of the website
Different Style resources
Inline Styles
Styles are applied directly to an HTML element using the style
attribute. These styles have the highest specificity and override other styles.
<p style="color: red; font-size: 18px;">This is a red, larger text using inline style.</p>
Internal Stylesheet
Styles are defined within a <style>
element in the HTML document. These styles apply to the entire document.
<head>
<style>
p {
color: blue;
font-size: 16px;
}
</style>
</head>
External Stylesheets
Styles defined in external CSS files linked to the HTML document using the <link> element. These stylesheets are loaded in the order they appear in the document and override previous styles if they have equal specificity.
<head>
<link rel="stylesheet" href="./styles/index.css" text="text/css" />
</head>
Task: Create a style folder inside your project directory name it styles, and we’ll create CSS files for each major section in this folder.
CSS Selectors and Properties
Two main components - Selectors & Properties
- Selectors work with identifiers in HTML, IDs, Classes, Element names
- Properties are assigned to those selectors for their respective elements.
These are some primary selectors we are going to use.
-
Tag Selector (
element
): Selects all instances of a specific HTML element, by their tag name.button { color: blue; background-color:#ffffff; }
- Class Selector (
.class
): Targets all elements with a specific class attribute.
.container { margin: 0; padding: 2rem; }
- ID Selector (#id): Targets a single element with a specific ID attribute.
#about { text-align: center; }
- Descendant Selector (ancestor-descendant): Targets elements nested within another.
ul li { list-style-type: square; } header img { width: 100px; }
- Child Selector (parent > child): Targets immediate or direct child elements of a parent element.
header > img { width: 100px; }
- Attribute Selector: Selects elements based on their attributes.
input[type="text"] { border: 1px solid #FFFFFF; }
- Pseudo-class Selector: Selects elements based on their state or position.
a:hover { text-decoration: underline; }
- Universal Selector: Selects all elements on a page.
* { margin-right: 0; padding-top: 0; }
- Grouping Selector: Groups multiple selectors to apply the same styles.
h1, h2, h3 { font-family: Arial, sans-serif; }
CSS Box Model
The CSS box model describes how elements on a web page are rendered in rectangular boxes. Think of it as the structure of each individual HTML element.
It consists of several components:
- Content: The actual content of the element, such as text or images.
- Padding: The space between the content and the element's border.Padding can be customized using the
padding
property. - Border: The border surrounding the padding and content.The border can be styled using the
border
property. - Margin: The space outside the border, creates separation between elements.Margins can be adjusted using the
margin
property.
Example from the website to understand border box.
Border Box vs. Content Box:
- By default, elements follow the "content-box" sizing, where the width and height only include the content area.
- You can use the
box-sizing
property to change the sizing model. For example,box-sizing: border-box
includes padding and border within the element's dimensions, making layout calculations easier.
Key Features of the CSS
Sizing Units
Absolute Units
These are fixed absolute units.
- Pixels (px): A single, fixed-size pixel on the screen. Commonly used for precise control over element dimensions.
- Inches (in): Represents a physical inch. Rarely used in web design due to the varying pixel densities of screens.
- Centimeters (cm): Represents a physical centimeter. Like inches, it's rarely used in web design for the same reason.
Relative Units
They can be relative to
- Percentage (%): Relative to the parent element's size. For example, setting
width: 50%
makes the element half the width of its parent. - Em (em): Relative to the font size of the element itself. If the font size of an element is 16px,
1em
is equal to 16px. It's useful for responsive typography. - Rem (rem): Relative to the root element's font size (usually the
<html>
element). It provides a consistent base for relative sizing throughout the page. - Viewport Units (vw, vh, vmin, vmax): Relative to the size of the viewport (the visible part of the web page). For example,
1vw
is 1% of the viewport width.
CSS Cascading and Specificity
Specificity
It determines which style is more specific and thus gets applied. The order of specificity,
IDs name > Class names > Element name
To understand this, imagine a <div>,
<div class="box" id="box1">
</div>
Writing border property for this div
#box1{
border: solid 1px green;
}
.box{
border: solid 1px red;
}
div{
border: solid 1px blue;
}
Despite that the blue border is defined at last, green border is going to be applied.
Note:
The different style resources (external stylesheets, inline styles, internal styles) also have a specificity order.
Inline Styles style
attribute >> Internal Stylesheet <style>
>> External Stylesheet
Cascading
Cascading is about the order in which styles are applied. Later rules override the earlier ones.
When multiple values are given for the same property on the same element, the last property: value is applied.
#box1{
border: solid 1px green;
border: solid 1px pink;
}
It applies to different stylesheets as well. The CSS files are loaded in the order they are added to the HTML document. So the last file’s styles are going to override the previous file styles if they have equal specificity.
Layout and Positioning
As the default layout provided by HTML is not enough, CSS provides you more control over the layout, positioning, and alignment of the elements on the webpage.
There are two CSS models or techniques:
- CSS Flexbox: A one-dimensional layout model that helps align and distribute items within a container, in a single direction, verticle or horizontally.
- CSS Grid: A two-dimensional layout model that creates a grid system for precise control over the placement and alignment of elements in both rows and columns. Preferred for complex layouts.
Task: Create a CSS file name global.css inside the style folder, we will start with global CSS, which applies to the entire document irrespective of the section.
Global.css
/* Variables */
:root {
--night-black: #1c0c1c;
--pitch-black: #180c14;
--primary-color: #612dbc;
--secondary-color: #66456a;
}
body {
font-family: "Lucida Sans", "Lucida Sans Regular", "Lucida Grande";
background-color: var(--pitch-black);
color: #fff;
margin: 0;
padding: 0;
font-size: 16px;
}
Example from the website:
To convert a container into a flex container:
Flexbox row
Flexbox column
Components of Flexbox and the properties associated with them:
Flex container
- The parent element holds a set of child elements and defines the context for the flex layout.
- Properties:
display: flex
ordisplay: inline-flex
to create a flex container or an inline-flex container, respectively.
Items (Flex Items):
- The child elements within the flex container that are laid out using the flex layout.
- Each item can grow or shrink to occupy available space according to the flex properties.
- Properties:
flex-grow
,flex-shrink
, andflex-basis
control item sizing within the container.
Main Axis:
- The primary axis along which flex items are distributed within the flex container.
- Controlled by the
flex-direction
property, which can be set torow
,row-reverse
,column
, orcolumn-reverse
.
Cross Axis:
- The perpendicular axis to the main axis, is used for aligning flex items.
- The direction is determined by the
flex-direction
property (opposite of the main axis).
Justify Content:
- Determines how flex items are spaced along the main axis.
- Options include
flex-start
,flex-end
,center
,space-between
, andspace-around
.
Align Self:
- Overrides the alignment set by
align-items
for individual flex items.
Flex Wrap:
- Determines whether flex items should wrap onto multiple lines if they overflow the container.
- Options are
nowrap
(default),wrap
, andwrap-reverse
.
Align Items:
- Controls how flex items are aligned along the cross axis.
- Options include
flex-start
,flex-end
,center
,stretch
, and more.
Order:
- Specifies the order in which flex items appear along the main axis.
Task: Let’s add the style to the individual sections of our portfolio.
Navbar
Without CSS, the logo and navigation elements are stacked inside the navbar.
Styling the navbar
- convert the navbar container into a flexbox
- space out the logo and the navigation elements
- style the ul
- style the list items li
- style the navigation links using pseudo-classes hover and active
Task: Create an index.js file to import and link all CSS files to the document.
@import "./global.css";
@import "./nav.css";
@import "./header.css";
@import "./about.css";
@import "./work.css";
@import "./skills.css";
@import "./contact.css";
@import "./footer.css";
Hero Section
- The hero section is a row flexbox with two main child, a textbox, and the hero image
- Convert the hero container into Flexbox
- convert the hero-text container into a flexbox to be able to align the items better
- style the hero image
- style the buttons inside global.css
About
- Two blocks
- the second block is a flexbox row, with two items: about-img and about-details
Portfolio Showcase
- The portfolio section contains two block-level elements - Heading, project box
- The project box is a container that holds all of the project cards
- project card is a flexbox row, which contains the project overview image and the details about it
- project-card-text which shows the project summary and the links to the code and live website, because images are inline elements they align on the same line, but if you turn them into links by wrapping the image with <a>, they’ll be block level elements, you’ll need to set display:inline;
Skillset
Although the skillset container is going to be a row flexbox, there are multiple lines of items, that we achieve using flex-wrap, which basically means wrapping/adjusting that one-directional row into multiple lines.
Contact
- Inside the main contact section, let’s focus on the second block which is a form.
- Convert the form into a verticle flexbox for better alignment, inside which there are multiple <div> containing the label and the corresponding input, and lastly a button.
- Each div inside form is a row flexbox, to align and space out the input and the element on the same line.
- Add box-shadow to the input on different states
Footer
- Two blocks, both <div>, one for socials and another one for address or any other footer info
- Convert Socials into a row flexbox, and the address into a column flexbox