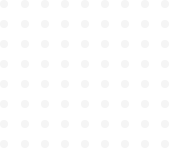
ReactJS project based training with Road Trip Planner App
Introducing and Integrating React Router
React Router
⚠️dependency: For adding subroutes to a react application we use a library called react-router. React provides seperate routing libraries for both web apps and native apps. As we are working on a web app, use npm i react-router-dom inside your terminal, to install react-router for dom.
A brief overview of React-router-dom and its components:
BrowserRouter: It is the top-level component of react-router-dom, keeps track of the current address, and takes care of navigation back and forth using the browser’s built-in history API.
Routes: Under BrowserRouter, we can have multiple <Routes/> but here we have just one route and two subroutes.
Route: <Route/> is to be used inside <Routes/> to create subroutes, and they can be nested as well.
Link: The Link element lets the user navigate to the routes and subroutes within an application. It’s almost like an <a/>
Inside your app component,
import React from "react";
import Home from "./pages/Home";
import "./utilities.css";
import Planning from './pages/planning';
import { Route, Routes, BrowserRouter as Router } from "react-router-dom";
const App = () => {
return (
<Router>
<Routes>
<Route path="/home" element={<Home />} />
<Route path="/plan" element={<Planning />} />
</Routes>
</Router>
)
}
export default App;
app.js
We added a router, and we have the routes and subroutes ready, now let’s create the links to these subroutes.
Logo should navigate to the home page,
Logo
import { ImLocation } from "react-icons/im";
import { Link } from "react-router-dom";
const Logo = () => {
return (
<Link to="/home" className="links">
<span className="Logo">
<ImLocation />
TheTrips.com
</span>
</Link>
);
};
export default Logo;
Logo.jsx
StartButton should navigate to the plan page,
StartButton
import { FiArrowRight } from "react-icons/fi";
import { Link } from "react-router-dom";
const StartButton = () => {
return (
<Link to="/plan" className="links">
<button className="start-button">
<p style={{ margin: "0 0.3rem" }}>Start</p>
<span>
<FiArrowRight size="1.2rem" />
</span>
</button>
</Link>
);
};
export default StartButton;
StartButton.jsx
Important Note: Inside your package.json you need to mention that your home page route is “/home” now, as by default it is “/”
And we are done!
Check out your localhost website and you should see that Home and Plan are two different subroutes now, on running the dev server you’ll land on the home page which is /home in our case. To navigate to the Plan page, click on the start button on the home page, and it will take you to the http://localhost:3000/plan.