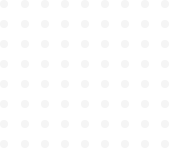
ReactJS project based training with Road Trip Planner App
ReactJS Environment setup
Project environment setup for ReactJS
We are gonna be writing a road trip app, I’ll call it TheTrips.com, you call it whatever you want. Let’s set up the project directory for the road trip app.
Note: Altho this is a quite simple process, and you’ll be done in two simple steps, we’ll take some time to understand and appreciate the need for the main tools involved in the setup.
I personally had a lot of questions while I was first learning all this, so I’ll try to share whatever I figured out, feel free to pass if you already know something :)
NodeJS
You must have heard about NodeJS by now, but still, here’s a brief introduction:
Node.js is a cross-platform, Javascript runtime environment, and a server-side platform.
Let's break it down,
cross-platform: you can run it on any machine Linux, windows, macOS, etc, and using it you can build applications for any machine, windows, mobile, Linux, and macOS
Javascript runtime environment: think of it as a box you can use to run javascript outside of the browser, but internally it uses the same V8 engine that Chrome uses.
server-side platform: altho Nodejs is used on frontend as well, it is called a server-side platform because it is used as a platform to host and run web servers
A little Story about NodeJS, earlier javascript was limited to only browsers before NodeJS, but now you can run Javascript code outside of the browser as well, and not only that Javascript was also limited to the client side for a long time and it was not possible for developers to write both frontend and backend in the same language, developers of NodeJS made it possible. And lastly, as NodeJS runs on Google Chrome’s V8 engine, which makes it very performant and hence a popular tool for every kind of project.
As NodeJS runs Javascript code on computers it has no concept of DOM, but yeah it provides other useful API
Now, here’s an interesting question that might cross your mind in good times
What’s the need for NodeJS when you’re supposed to run your React web app on a browser only?
Here’s why,
For working with React you need to install the core React libraries/packages, react, and react-dom. We install these packages using npm or yarn, and both can only work alongside NodeJS.
Just FYI, Rather than installing these packages locally, you can also use them directly from CDN, by loading it using <script/> tag like this,
<script crossorigin src="<https://unpkg.com/react@18/umd/react.production.min.js>"></script>
<script crossorigin src="<https://unpkg.com/react-dom@18/umd/react-dom.production.min.js>"></script>
unpkg is the CDN for NPM, react@18 means version 18 of react, and …min.js is nothing but minified code.
But even if you decide to load these using CDN and not npm, you will need to use npm to install the build tools like webpack or babel and other third-party libraries for your project. Basically, modern web development is almost impossible without Node. Any bundler or CSS framework, require the node to run on.
Hopefully, I’ve done a good job selling Node to you! :)
STEP-1: Install NodeJS from here
NPM
Not to confuse you, but npm is two things :
The world's largest software registry, where people can upload and download packages, is called NPM.
A package manager for Node is also called npm, it installs packages from the NPM registry, and manages them (like updating, etc ) for your project.
NPM comes with Node JS, so when you install Node JS, you should have npm installed.
node_modules folder
Every npm project has a node_modules folder. NPM installs these packages locally and globally under node_modules. By default, they are installed locally under node_modules in the current directory of the project.
package.json
You might have noticed a package.json file inside your root directory, whenever npm installs a package, it adds the name and the version of the package to the dependencies property inside the package.json.
"dependencies": {
"react": "^18.2.0",
"react-dom": "^18.2.0",
}
// the ' ^ ' is to denote package version greater than or equal to
Why need a package.json file?
It holds metadata for the Node/npm project, and it really is the core of your project. For now, you just need to know that npm uses a package.json to install dependencies - suppose you published this project on GitHub, and now your friends want to check out your project by running it on their machine, but for that, they need to install all the packages you used for building this project - just by typing npm install they have it all set. NPM will install all of those packages with all the correct versions for you.
Dependencies: all the packages your project is dependent on
package-lock.json
In simple terms, this file is to lock the versions of dependencies. Package.json contains ranges for packages like “^8.0.2” which means versions 8.0.2 and above, which means it records the minimum version required. But the package-lock.json file records the exact same version of the package that the original project was built with. So your friend has to use the command npm ci to install all the exact same versions you used from package-lock.json.
STEP-2: Now that you have Node and npm installed, set up your project directory
Inside VScode open any folder where you want to create your project folder, go to the Terminal tab and click on New Terminal
Inside the Terminal, execute this code
npx create-react-app roadtrip
This will create a new folder named myroadtrip inside your current directory, like in my case it’s code. To navigate to your project folder, execute this below line and it will take you to your project directory,
Create-react-app
It is a tool to create single-page frontend applications, it doesn’t handle the backend or storage. You should have npm and node installed and with a single command, you will have a beginner-friendly development environment ready, with all required configurations.
npx create-react-app roadtrip
cd my-app
npm start // it runs your react app on your default browser
NPX: Node package execute (npx) is the npm package runner, it executes the npm packages directly from the Npm registry, without locally installing and storing them. You don’t need to install it, it comes with npm. We need to use create-react-app only once and then never, so there’s no point in storing it locally.
It will create a folder name roadtrip and treat it as the root folder.
Create-react-app uses a few tools inherently to manage some things, you don’t need to deal with them directly, but it’s better to have an idea of what is going under the hood. Let’s see.
Webpack
Webpack is a module bundler.
from webpack
What it is?
Module bundler: Basically a module bundler bundles all your javascript files and their dependencies in a single javascript file, Webpack names it bundle.js.
But create-react-app
Babel
It is a javascript transpiler, to translate modern javascript code into backward compatible javascript code, it basically means that you can write javascript code with any latest syntax, and babel lets you run it on browsers that do not support this syntax.
For example, some old browsers might not support javascript ES6 or later versions, babel will convert your ES6 code into ES5 code before it is bundled, so now your code will run on that older browser as well.
If you further want to know how create-react-app uses Babel under the hood, you’ll find about it inside the webpack.config that we saw inside /node_modules/react-scripts/config/webpack. Webpack uses babel as a loader.
Adding CSS
Although, there are many ways to add styling to the components like CSS Modules, importing stylesheets, inline CSS, and using libraries like styled components, etc. But in this project, we will keep it simple by creating .css files like you usually do in a vanilla javascript project, but instead of linking it inside the index.html file, we import it inside React files.
import "./home.css";
We can either create a huge CSS file and import it inside every single component module, or make our life easier by creating a CSS file for every single component separately, and a global CSS file that contains the styles used by all of the modules like buttons, headings, body, etc. I have named it utility and imported it way up in our component hierarchy, i.e inside <App/>