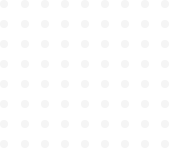
ReactJS project based training with Road Trip Planner App
Introducing Local Storage
Now try interacting with the DestinationForm component, and the input fields, see what happens and what should happen, and try to figure out the events that should take place.
Let’s start with the input elements, when you enter the name of a location, date, and time, it should all reflect inside a card on the TripBoard, right?
Not only when a user enters this information, but also after a refresh it should all be there. For this, we need to store this information somewhere but we don’t wanna go on to the backend yet so we’ll make do with the local storage for now.
And what exactly are we going to store?
whenever a user enters a location, it’ll get added to an array called locations, as an object with properties id, name, date and time. Remember the locations array from the demo_props we used,
"locations" : [
{ id: 742, name: "Jaipur, Rajasthan", date: "2022-12-20", time: "07:30" },
{ id: 489, name: "Jodhpur, Rajasthan", date: "2022-12-23", time: "10:00" },
{ id: 201, name: "Alwar, Rajasthan", date: "2022-12-28", time: "08:55" },
],
This is data we need to store inside local storage, let’s understand the localStorage now,
localStorage: It’s a part of the Web Storage API, this API allows you to store data inside the user’s browser. Web storage provides two mechanisms and their respective objects for storing data on the client side:
- window.localStorage
- window.sessionStorage
You can check it out by going inside the dev tools, and from there to the Application Panel on the tab menu,
👆 Step-1
👆 Step-2
The localStorage allows data to retain even after the page has been reloaded. localStorage is an object representing local storage, it stores data just like any other object in javascript, as key-value pairs. But unlike normal javascript objects, it provides two methods to store and retrieve data, which are
- setItem()
- getItem()
Let’s discuss the usage of these methods before moving on,
localStorage.setItem(key, value)
As I said earlier localStorage stores data in key-value pairs, both the key and the value is of type string, in our case for key we will pass “locations” and for the value, we need to convert the array into a string using another method JSON.stringify()
localStorage.setItem("locations", JSON.stringify([
{ id: 742, name: "Jaipur, Rajasthan", date: "2022-12-20", time: "07:30" },
{ id: 489, name: "Jodhpur, Rajasthan", date: "2022-12-23", time: "10:00" },
{ id: 201, name: "Alwar, Rajasthan", date: "2022-12-28", time: "08:55" },
]));
localStorage.getItem(key)
Because instead of an array we stored the stringified version of the array, this method is going to return that string only, so we are supposed to convert it back into an array, for that, we use the method JSON.parse()
JSON.parse(localStorage.getItem("locations"))