File Handling in PHP
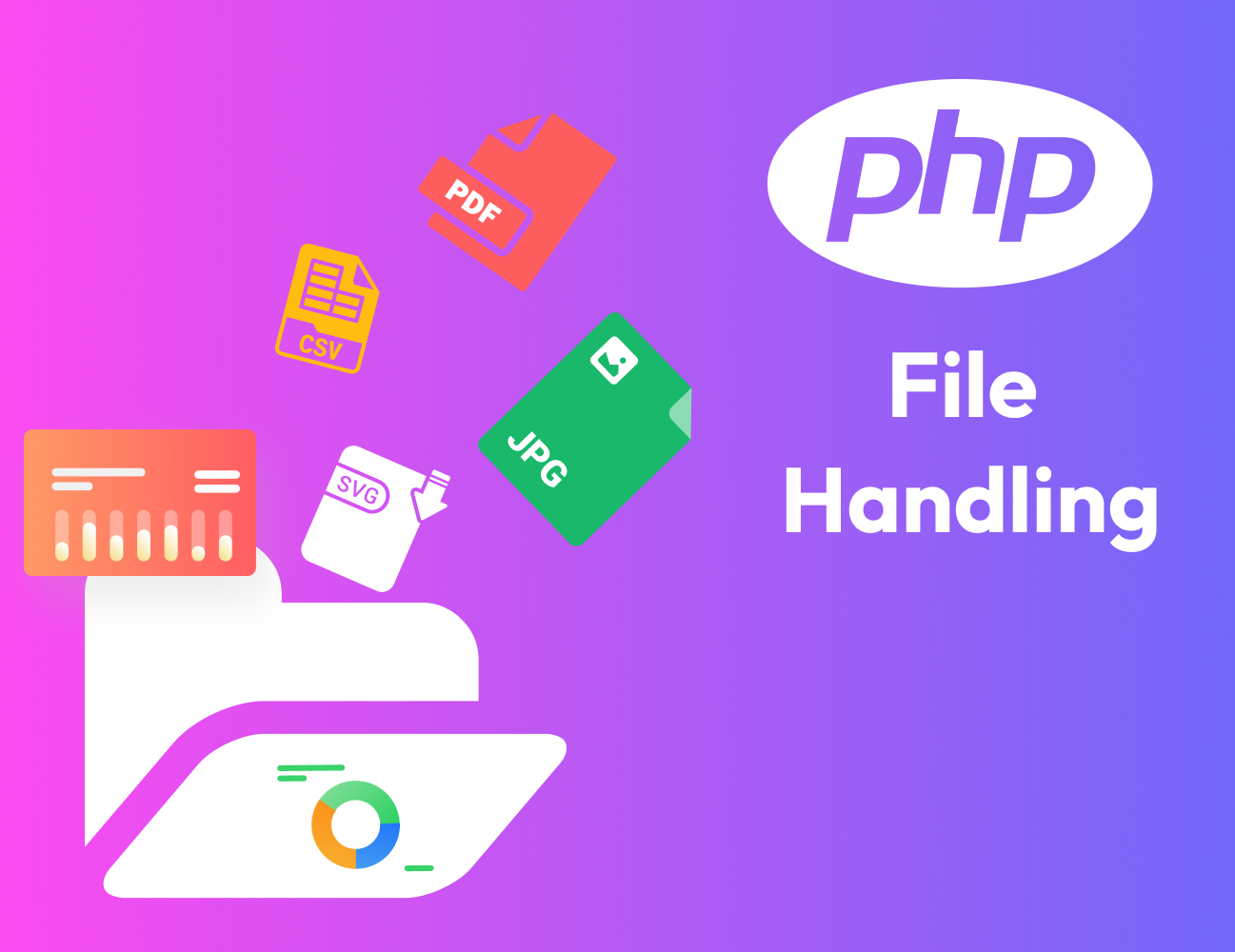
Prerequisite: Basic Understanding HTML, CSS, PHP and MySQL.
In this project:
- We will create an HTML Form.
- A dropdown menu will be created to perform following file functions:
- Read
- Write
- Append
- Delete
- A textfield to insert the filename on which the operation will be performed.
- A textfield to insert or display file data.
- All the files are stored inside the files folder under same directory.
index.php ⬇
<?php
$taskArr = array('Read', 'Write', 'Append', 'Delete');
if (isset($_POST['filename']) && $_POST['filename'] != "" && isset($_POST['submit'])) {
$filename = $_POST['filename'];
$error = $text = "";
if(!strstr($filename, '.txt')){
$filename = $filename.".txt";
}
if(isset($_POST['text']) && $_POST['text'] != ''){
$text = trim($_POST['text']);
}
$task = $_POST['action'];
switch($task) {
case 'Read':
if(file_exists("files/".$filename)){
$pointer = fopen("files/".$filename, 'r');
$text = fread($pointer, filesize("files/" . $filename));
fclose($pointer);
}else{
$error = "<p class='error'>File does not exist.</p>";
}
break;
case 'Write':
if(file_exists("files/" . $filename)){
$error = "<p class='error'>File name already exists.</p>";
}else{
$pointer = fopen("files/" . $filename, 'w');
fwrite($pointer, $text);
fclose($pointer);
}
break;
case 'Append':
if(file_exists("files/" . $filename)){
$pointer = fopen("files/" . $filename, 'a');
fwrite($pointer, $text);
fclose($pointer);
}else{
$error = "<p class='error'>File does not exist.</p>";
}
break;
case 'Delete':
if(file_exists("files/" . $filename)){
unlink("files/" . $filename);
$error = "<p class='success'>File deleted.</p>";
}else{
$error = "<p class='error'>File does not exist.</p>";
}
break;
}
}
?>
<!DOCTYPE html>
<html>
<head>
<title>File Handling</title>
<link rel="stylesheet" href="https://maxcdn.bootstrapcdn.com/bootstrap/3.3.7/css/bootstrap.min.css">
<script src="https://ajax.googleapis.com/ajax/libs/jquery/3.5.1/jquery.min.js"></script>
<style>
.error{color: red} .success{color:green}
</style>
</head>
<body>
<div class="col-md-4">
<form action="" method="post">
<table align="center" class="table">
<tr>
<td>SELECT TASK</td>
<td>
<select name="action" class="form-control" onchange="msgbox(this)">
<option value="">-- SELECT --</option>
<?php
foreach($taskArr as $val){
$selected = '';
if(isset($task) && $task != '' && $val == $task){
$selected = " selected='selected'";
}
echo '<option value="'.$val.'" '.$selected.'>'.$val.'</option>';
}
?>
</select>
</td>
</tr>
<tr>
<td>FILE NAME</td>
<td>
<input type="text" name="filename" class="form-control" value="<?= (isset($filename) && $filename != '') ? $filename : ''; ?>">
</td>
</tr>
<tr>
<td>FILE TEXT</td>
<td id="textareaDiv">
<?php
if(isset($error) && $error != ''){
echo $error;
}else{
echo '<textarea name="text" rows="12" cols="35" class="form-control">';
if(isset($text) && $text != ''){
echo $text;
}else{
echo '';
}
echo '</textarea>';
}
?>
</td>
</tr>
<tr>
<td colspan="2">
<input type="submit" name="submit" value="SUBMIT" class="btn btn-primary">
</td>
</tr>
</table>
</form>
</div>
<script>
function msgbox(obj){
if(obj.value == 'Write' || obj.value == 'Append'){
$("#textareaDiv").html('<textarea name="text" rows="12" cols="35" class="form-control"></textarea>');
}else{
$("#textareaDiv").html("");
}
}
</script>
</body>
</html>
Run index.php file in your browser to get the following output.
Code Explanation of index.php
include_once 'includes/conn.php';
- Connection file is included which includes the query to connect to the database.
DESCRIPTION
$taskArr = array('Read', 'Write', 'Append', 'Delete');
Values for select box of file handling actions are taken in a task array.
SELECT TASK
<select name="action" class="form-control" onchange="msgbox(this)">
<option value="">-- SELECT --</option>
<?php
foreach($taskArr as $val){
$selected = '';
if(isset($task) && $task != '' && $val == $task){
$selected = " selected='selected'";
}
echo '<option value="'.$val.'" '.$selected.'>'.$val.'</option>';
}
?>
</select>
-
Selectbox with name=”action” is taken.
-
Array
$taskArr
is traversed to display the values in this selectbox. -
If
$task
variable is already set i.e. some action is already posted by the form, that particular action value will be selected automatically.
FILE NAME
<input type="text" name="filename" class="form-control" value="<?= (isset($filename) && $filename != '') ? $filename : ''; ?>">
-
An input field with name=”filename” is created for user to enter the name of file.
-
If any filename is already posted, it will be displayed in the input field.
FILE TEXT
<div id="textareaDiv">
<?php
if(isset($error) && $error != ''){
echo $error;
}else{
echo '<textarea name="text" rows="12" cols="35" class="form-control">';
if(isset($text) && $text != ''){
echo $text;
}else{
echo '';
}
echo '</textarea>' ;
}
?>
</div>
-
“textareaDiv”
block is created to display the error messages or the text of the files, whichever is supposed to.
OUTPUTS RELATED TO ABOVE EXPLANATION IS AS UNDER:
$filename = $_POST['filename'];
if(!strstr($filename, '.txt')){
$filename = $filename.".txt";
}
- If in case user has entered the filename without extension, the extension is appended with the filename.
$task = $_POST['action'];
switch($task) {
…….
}
- User selected action is passed to the switch statement where all the cases are handled separately.
case 'Read':
if(file_exists("files/".$filename)){
$pointer = fopen("files/".$filename, 'r');
$text = fread($pointer, filesize("files/" . $filename));
fclose($pointer);
}else{
$error = "<p class='error'>File does not exist.</p>";
}
break;
In case of READ,
if(file_exists("files/".$filename)){
}
- If the file exists, the control is passed to the if block. Otherwise, error message is displayed in else block.
$pointer = fopen("files/".$filename, 'r');
- File is opened in READ mode by mentioning the required path of file.
$text = fread($pointer, filesize("files/" . $filename));
- File is read and the content is stored in $text variable and is echoed in textarea.
case 'Write':
if(file_exists("files/" . $filename)){
$error = "<p class='error'>File name already exists.</p>";
}else{
$pointer = fopen("files/" . $filename, 'w');
fwrite($pointer, $text);
fclose($pointer);
}
break;
In case of WRITE
- If file exists,
$error
variable is assigned with an error message. - Else, File is opened in WRITE mode using
fopen()
method. $text
variable values are written inside the file usingfwrite()
method.- file is closed using
fclose()
method.
case 'Append':
if(file_exists("files/" . $filename)){
$pointer = fopen("files/" . $filename, 'a');
fwrite($pointer, $text);
fclose($pointer);
}else{
$error = "<p class='error'>File does not exist.</p>";
}
break;
In case of APPEND,
- if file exists, it is opened in an append mode using
fopen()
method and passing ‘a’ as second parameter where a stands for append. fwrite()
method is used to append $text content at the end of the file.- File is closed using
fclose()
method
case 'Delete':
if(file_exists("files/" . $filename)){
unlink("files/" . $filename);
$error = "<p class='success'>File deleted.</p>";
}else{
$error = "<p class='error'>File does not exist.</p>";
}
break;
In case of DELETE,
- If file exists, it is deleted using
unlink()
method. - Else,
$error
variable is assigned with a required error message.
Summary
In this project, we have learned all file handling operations like read, write, append and delete and are implemented using SWITCH statement in a single program using PHP.