Generate PDF File of MySQL Data using PHP mPDF Library
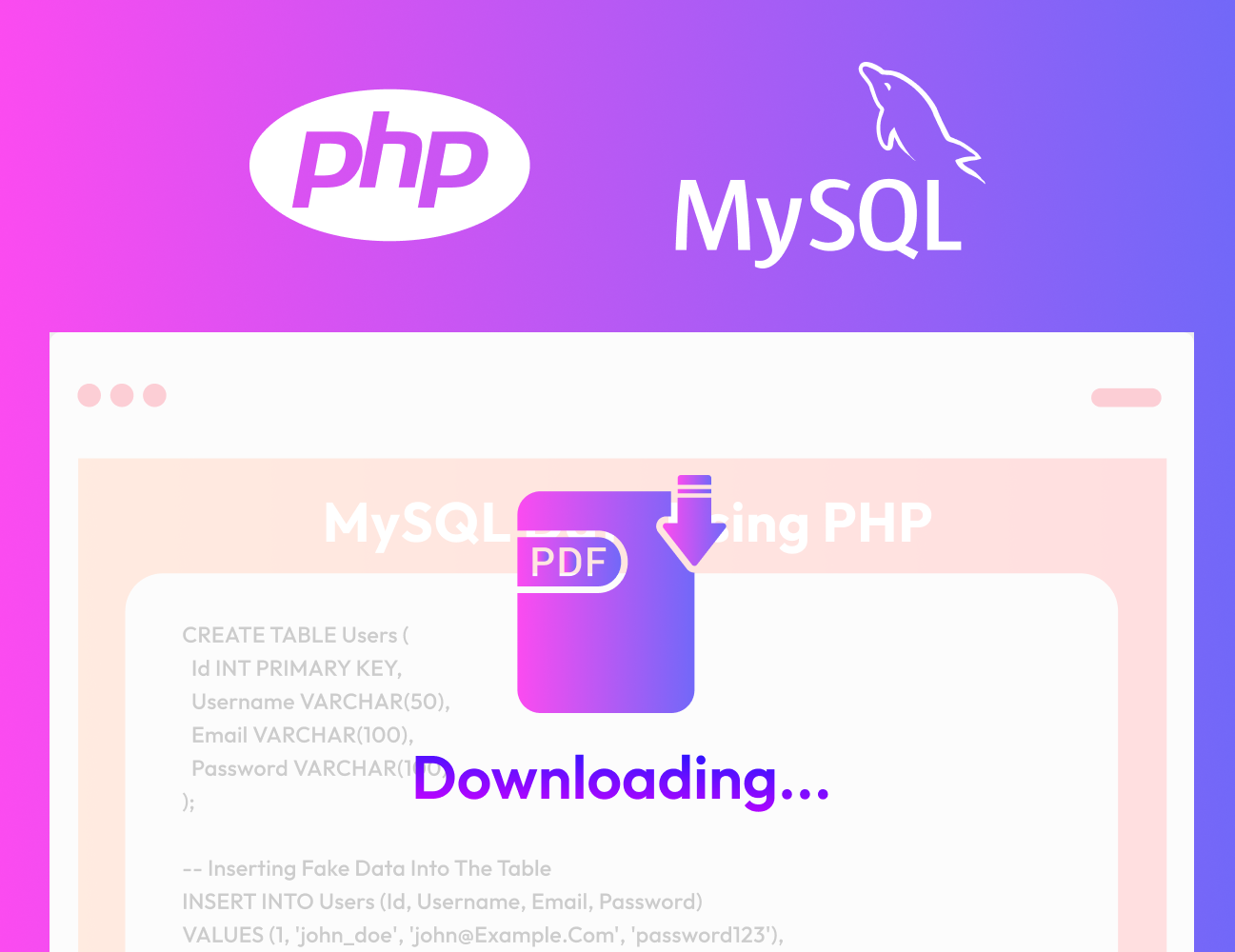
Prerequisite: Basic Understanding HTML, PHP and MySQL.
PDF is a Portable Document Format that cannot be manipulated by the users. PDFs are generated by various organizations on daily basis for example : to generate a invoice, receipts and the student report cards, identification cards etc.
PHP Libraries are there to generate PDFs on click. For an instance: FPDF, mPDF, DOMPDF etc. We shall be using mPDF Library to create PDF of MySQL Data using PHP. mPDF is a PHP Library which is used to generate a PDF File from UTF-8 encoded HTML. It is an advanced version of FPDF with multiple number of enhancements. This Library is written by LAN BACK and was released under GNU GPL v2 License. Various features of this library are:
- Page Numbering
- Colours
- Bookmark
- Annotation
- Page Header and Footer
- HTML Support
- CSS Stylesheets
In this project:
- We will download and install the mPDF library
- mPDF Library files will be included in our main PHP file.
- MySQL connection file is also included in this file to fetch the required data from the table.
- We shall be using mPDF inbuilt methods to set the HEADER and FOOTER of PDF pages.
- Data is also displayed in HTML form using the required method of mPDF Library.
Index.php⬇
<?php
require_once __DIR__ . '/vendor/autoload.php';
include_once 'includes/conn.php';
$mpdf = new \Mpdf\Mpdf();
$mpdf->SetHTMLHeader('<h3>USER DETAILS</h3>');
$html = '<table width="100%" border="1" cellspacing="0" cellpadding="10">
<tr>
<th>Name</th>
<th>Email ID</th>
<th>Department</th>
<th>Salary</th>
</tr>
';
$sql = "SELECT * FROM users a LEFT JOIN user_details b ON a.user_id = b.user_id WHERE a.status = 1";
$exe = mysqli_query($con, $sql);
$res = mysqli_num_rows($exe);
if($res > 0){
while($record = mysqli_fetch_assoc($exe)){
$html .= "<tr>";
$html .= "<td>".ucfirst($record['name'])."</td>
<td>".$record['email']."</td>
<td>".$record['department']."</td>
<td>".$record['salary']."</td>";
$html .= "</tr>";
}
}else{
$html .= "<tr>
<td colspan='4' style='color:red; text-align:center'>No Record Found</td>
</tr>";
}
$html .= "</table>";
$mpdf->WriteHTML($html);
$mpdf->SetHTMLFooter('<table width="100%">
<tr>
<td width="50%">Date: {DATE j-m-Y}</td>
<td width="50%" align="right">Page {PAGENO}/{nbpg}</td>
</tr>
</table>');
$mpdf->AddPage();
$mpdf->Output();
When you run this index.php
file, PDF is generated and is displayed in browser as follows.
Important Instructions:
- while installing composer in windows the path of php is
`d:\xampp\php\php.exe`.
- Also enable gd extension in php.ini file
`d:\xampp\php\php.ini`
enable`extension=gd`
just remove `;` in front of this extension. - `composer require mpdf/mpdf` > if version of mpdf is less than 8 you can change version in composer.json file (
"mpdf/mpdf": "^8.0"
) and remove old vendor directory and - run this command again `composer install` then it will install latest version of mpdf.
Explanation of Index.php
- autoload.php file is used to autoload or declare all the required libraries for our project.
require_once __DIR__ . '/vendor/autoload.php';
$mpdf
is created as an instance of the Mpdf Class which is further used to access all the methods of this class.
$mpdf = new \Mpdf\Mpdf();
- PHP in-built method
include_once()
is used to include the connection file in our program which is used to create connection with mydb database.
include_once 'includes/conn.php';
- Connection is created with
“mydb”
database and in case of any error, Error message is displayed on the screen andexit()
method is called.Conn.php
file has the following set of code:
$con = new mysqli("localhost","root","","mydb");
if($con->connect_errno) {
echo "Failed to connect to MySQL: ". $con->connect_error;
exit();
}
- We have set the HEADER for all PDF pages using
SETHTMLHeader()
method.
$mpdf->SetHTMLHeader('<h3>USER DETAILS</h3>');
This header is automatically added to all the pages of a PDF Document.
- We have simple assigned a table row with carrying table headings to the
$html
variable as :
$html = '<table width="100%" border="1" cellspacing="0" cellpadding="10">
<tr>
<th>Name</th>
<th>Email ID</th>
<th>Department</th>
<th>Salary</th>
</tr>
';
- We have created
“users”
and“user_details”
table in the database and also we have entered some dummy records to fetch the data to generate PDF File. You can create the tables as per your own requirement. Our tables look like as:
users Table:
Where,
user_id
is the primary key of users table.
user_details Table:
Where,
Id is the primary key of user_details table and user_id acts as foreign key for this table and refers to primary key of users table.
LEFT JOIN is used to fetch the name, email, department and salary of all the users existed in the records.
- Records are fetched using
mysqli_query
, If the user records exists then the results are stored in$html
variable.
while($record = mysqli_fetch_assoc($exe)){
$html .= "<tr>";
$html .= "<td>".ucfirst($record['name'])."</td>
<td>".$record['email']."</td>
<td>".$record['department']."</td>
<td>".$record['salary']."</td>";
$html .= "</tr>";
}
- Write
HTML()
method is called by passing$html
variable as parameter to display the content in PDF Document.
$mpdf->WriteHTML($html);
SetHTMLFooter()
method is also used to define the footer of our PDF File. This footer will be displayed on all the pages of the PDF File. Footer can be created according the user requirement. Our footer is as:
$mpdf->SetHTMLFooter('<table width="100%">
<tr>
<td width="50%">Date: {DATE j-m-Y}</td>
<td width="50%" align="right">Page {PAGENO}/{nbpg}</td>
</tr>
</table>');
We have displayed the current date on the left bottom corner of the page and Page Number on the right bottom of the page. {PAGENO} is a variable that carries the value of current page of the PDF File.
AddPage()
method is called to add a new page to the PDF File.
$mpdf->AddPage();
Header and Footer is set automatically to the newly added page as well.
Output()
method is used to finalise the generated document and then display it to the web browser.
$mpdf->Output();
Summary
In this project, we have learned how to generate a PDF File using mPDF PHP inbuilt Library functions. It is installed by running instructions on the composer. Files are stored in our main directory and are included using php include method. PDF File data is created using in-built mPDF functions like SetHTMLHeader() is called by passing HTML header content as parameter to create a HEADER for PDF file.
In this project, we have fetched the MySQL Data and has generated a PDF file of that data. You can create a PDF of any kind of data as per your requirement.