Image Uploading in PHP
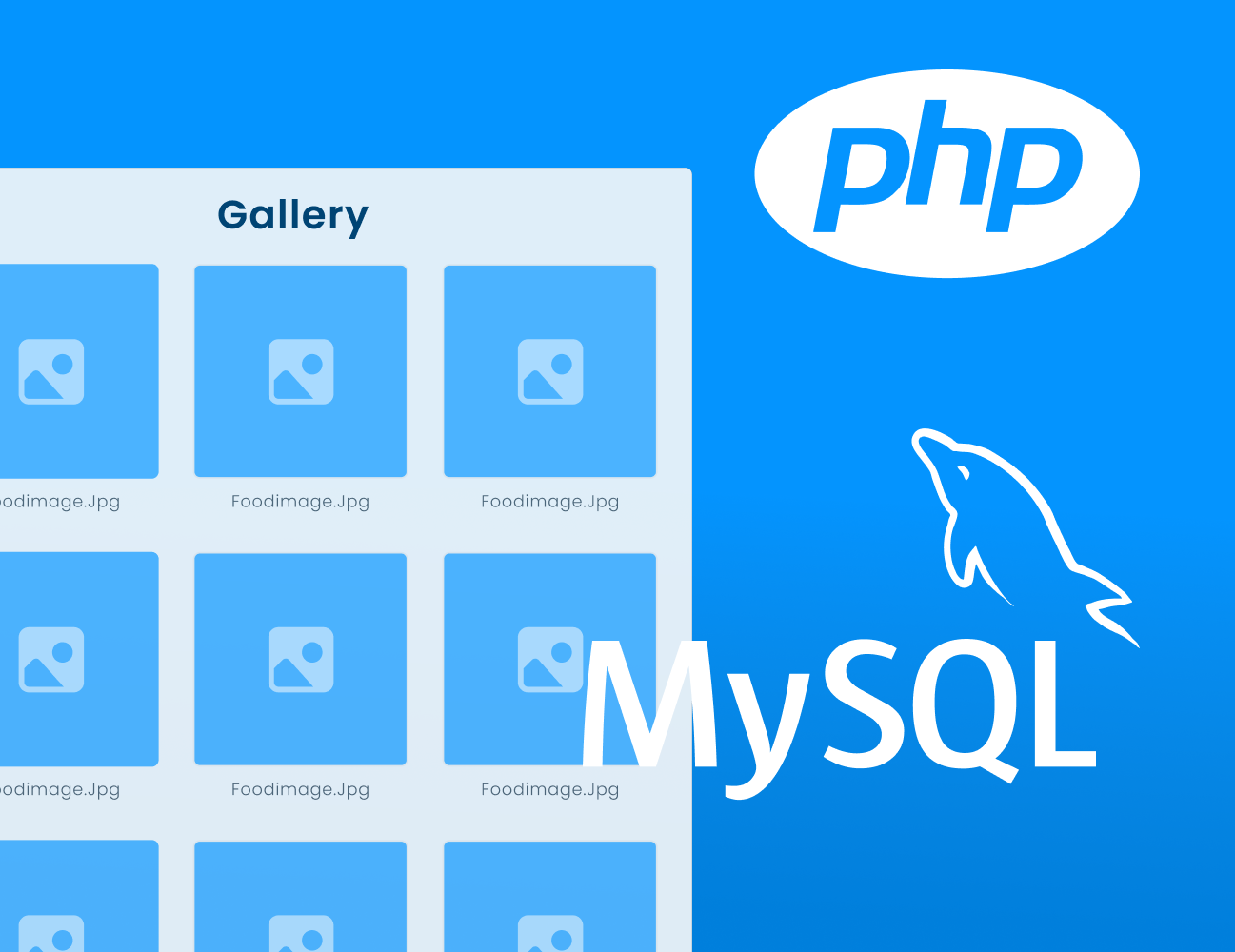
Prerequisite: Basic Understanding HTML, CSS, PHP and MySQL.
In this project:
- We will create an HTML Form.
- Upload an image from to the server directory using PHP.
- Inserting the name of the file in MySQL database, which is further used to retrieve that image from the directory.
index.php ⬇
<?php
include_once 'includes/conn.php';
$msg = "";
if (isset($_POST['upload'])) {
$filename = $_FILES["image"]["name"];
$tempname = $_FILES["image"]["tmp_name"];
$path = "images/".$filename;
$sql = "INSERT INTO upload(filename, created_at) VALUES ('$filename', '".date('Y-m-d H:i:s')."')";
mysqli_query($con, $sql);
if(move_uploaded_file($tempname, $path)){
$msg = "<p class='success'>Image uploaded successfully.</p>";
}else{
$msg = "<p class='failure'>Failed to upload image.</p>";
}
}
?>
<html>
<head>
<title>Image Upload</title>
<link rel="stylesheet" href="https://maxcdn.bootstrapcdn.com/bootstrap/3.3.7/css/bootstrap.min.css">
<style>
.wrapper{margin: 20px 50px;}
p{margin: 10px}
.success{color: green}
.failure{color: red}
</style>
</head>
<body>
<div class="wrapper">
<?php
if(isset($msg) && $msg != ""){
echo $msg;
}
?>
<form method="POST" action="" enctype="multipart/form-data">
<input type="file" name="image" value="" accept="image/*"><br>
<button type="submit" value="" class="btn btn-primary" name="upload">UPLOAD</button>
</form>
</div>
</body>
</html>
Run index.php file in your browser to get the following output.
Code Explanation of index.php
• Connection file is included which includes the query to connect to the database. DESCRIPTION
• A form is created having input type “file”. • •
|
DESCRIPTION• • <input type="file" name="image" value="" accept="image/*"> • • • • move_uploaded_file( this in-built method is used to move the image or file to the server. |
RENAME FILE IN DESTINATION FOLDER
We can also change the name of the file before saving it to the server. For Example:
$filename = $_FILES[‘image’][‘name’];
$temp = explode(‘.’, $filename);
$extension = end($temp);
$newfilename = time().”.”.$extension;
move_uploaded_file($_FILES[‘image’][‘tmp_name’], ‘images/’.$newfilename);
where, new filename will be the current timestamp and the extension of the image.
SIZE AND TYPE CHECK OF THE FILE TO BE UPLOADED IN DESTINATION FOLDER
We can apply a check for the size and the type of the file or image before uploading it to the server.
$filename = $_FILES[‘image’][‘name’];
$allowed_ext = array(“png”, “jpg”, “jpeg”);
$extension = pathinfo($filename, PATHINFO_EXTENSION);
$size = $_FILES[‘image’][‘size’];
if(!in_array($extension, $allowed_ext)){
$msg = “Only JPEG and PNG images are allowed”;
}elseif($size > 1000000){
$msg = “Size cannot exceed 1MB”;
}
- We can change the size and allowed extensions according to our requirement.
- pathinfo(
$filename
,PATHINFO_EXTENSION
), is an in-built method to return the extension of an image.
IF FILE ALREADY EXISTS CHECK WHILE UPLOADING
$file = “/images/”.$filename;
if(file_exists($file)){
$msg = “File Already Exists.”;
}
A PROGRAM TO UPLOAD AN IMAGE TO THE SERVER AND ALSO VALIDATING THE IMAGE.
- Size should not exceed 1MB
- Allowed extensions are JPG, JPEG and PNG
- If file already exists rename the file before saving it in the server
<?php
include_once 'includes/conn.php';
$msg = "";
if (isset($_POST['upload'])) {
$error = 0;
$filename = $_FILES["image"]["name"];
$tempname = $_FILES["image"]["tmp_name"];
$path = "images/".$filename;
$extension = pathinfo($filename, PATHINFO_EXTENSION);
// VALIDATIONS
$allowed_ext = array("png", "jpg", "jpeg");
$size = $_FILES["image"]["size"];
$file = "/images/".$filename;
if(!in_array($extension, $allowed_ext)){
$_SESSION['msg'] = "Only JPEG and PNG images are allowed";
$error = 1;
}elseif($size > 1000000){
$_SESSION['msg'] = "Size cannot exceed 1MB";
$error = 1;
}
if($error == 0){
if(file_exists($file)){
$filename = time().".".$extension; // rename if file already exists.
}
if(move_uploaded_file($_FILES['image']['tmp_name'], 'images/'.$filename)){
$sql = "INSERT INTO upload(filename, created_at) VALUES ('$filename', '".date('Y-m-d H:i:s')."')";
mysqli_query($con, $sql);
$_SESSION['msg'] = "<p class='success'>Image uploaded successfully.</p>";
}else{
$_SESSION['msg'] = "<p class='failure'>Failed to upload image.</p>";
}
}else{
$_SESSION['msg'] = "<p class='failure'>".$_SESSION['msg']."</p>";
}
}
?>
<html>
<head>
<title>Image Upload</title>
<link rel="stylesheet" href="https://maxcdn.bootstrapcdn.com/bootstrap/3.3.7/css/bootstrap.min.css">
<script src="https://ajax.googleapis.com/ajax/libs/jquery/3.5.1/jquery.min.js"></script>
<style>
.wrapper{margin: 20px 50px;}
p{margin: 10px}
.success{color: green}
.failure{color: red}
</style>
</head>
<body>
<div class="wrapper">
<div>
<?php
if(isset($_SESSION['msg']) && $_SESSION['msg'] != ''){
echo $_SESSION['msg'];
unset($_SESSION['msg']);
}
?>
</div>
<form method="POST" id="imageUploadForm" action="index.php" enctype="multipart/form-data">
<input type="file" name="image" value="" accept="image/*"><br>
<button type="submit" value="" class="btn btn-primary" name="upload" id="upload">UPLOAD</button>
</form>
</div>
</body>
</html>
Summary
In this project, we have learned to upload an image to the server directory and applying validations to check file or image before uploading it to the server using PHP.