Submit HTML Form Data using AJAX and send to an Email ID
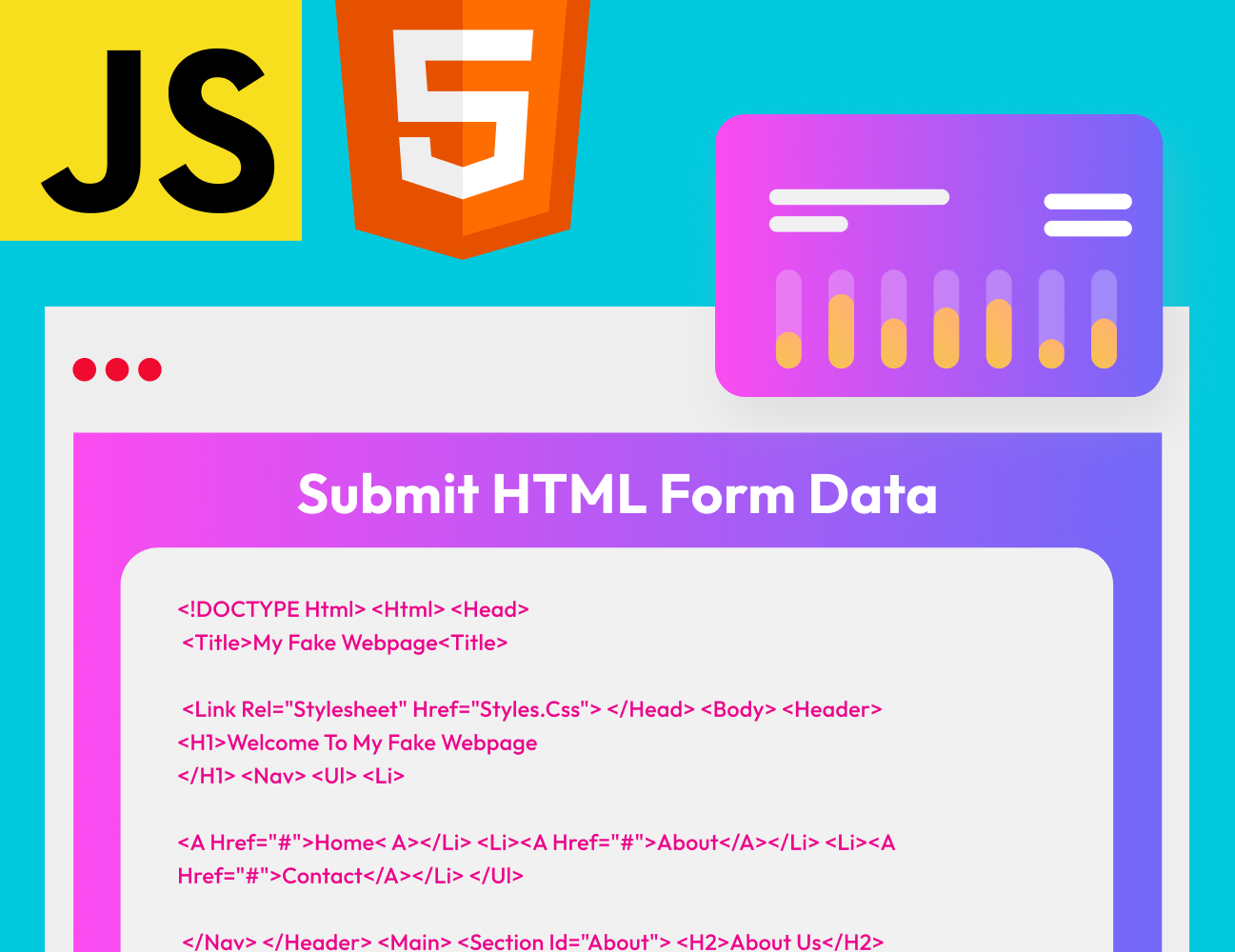
Prerequisite: Basic Understanding of HTML, CSS, JavaScript, jQuery, PHP and MySQL.
In this project:
- We will create a HTML Contact Us Form to take information from the user.
- The form consists of Name, Email, Phone, Subject and Message textfields and a “SEND MESSAGE” button.
- When the user clicks on the “SEND MESSAGE” button jQuery click function is called where ajax is called to process the form and email that form data to the specific email ID.
- Information is also stored in “contact_us” table using mysqli SQL statements for future reference.
- Incase of any error, error variable is set to 1 and msg is stored in
$arr[‘msg’]
variable and then is echoed after encoding it to the JSON array.
index.php ⬇
<!DOCTYPE html>
<html>
<head>
<title>Contact Us Form</title>
<link rel="stylesheet" href="https://maxcdn.bootstrapcdn.com/bootstrap/3.3.7/css/bootstrap.min.css">
<script src="https://code.jquery.com/jquery-3.5.1.min.js"></script>
<style>
.error{color: red; padding: 10px 0;}
</style>
</head>
<body>
<div class="container">
<h3>Contact Us</h3>
<form method="POST" action="" id="contact_form">
<div class="row">
<div class="col-md-4">
<label>Enter Name: </label>
<input type="text" name="name" class="form-control" id="name">
</div>
</div>
<div class="row">
<div class="col-md-4">
<label>Enter Email ID: </label>
<input type="email" name="email" class="form-control" id="email">
</div>
</div>
<div class="row">
<div class="col-md-4">
<label>Enter Phone: </label>
<input type="text" name="phone" class="form-control" id="phone">
</div>
</div>
<div class="row">
<div class="col-md-4">
<label>Subject: </label>
<input type="text" name="subject" class="form-control" id="subject">
</div>
</div>
<div class="row">
<div class="col-md-4">
<label>Message: </label>
<textarea class="form-control" cols="15" rows="5" name="message" id="message"></textarea>
</div>
</div>
<br>
<div class="row">
<div class="col-md-4">
<input type="hidden" name="action" value="submit_contact__form">
<button type="button" class="btn btn-primary" name="message_btn" id="message_btn">SEND MESSAGE</button>
</div>
</div>
</form>
</div>
<script>
$(document).ready(function(){
$("#message_btn").click(function(){
var name = $("#name").val();
var email = $("#email").val();
var phone = $("#phone").val();
var subject = $("#subject").val();
var message = $("#message").val();
if(name == "" || email == "" || phone == "" || subject == "" || message == ""){
alert("All fields are required.");
}else{
$.ajax({
url: 'process.php',
type: 'POST',
data: $("#contact_form").serialize(),
success: function(data){
var arr = JSON.parse(data);
alert(arr['msg']);
$("#contact_form")[0].reset()
},
error: function(){
}
});
}
});
});
</script>
</body>
</html>
- To use jQuery Functions jQuery file is included in the head tag. You can use either an online link or download it from the
jQuery.com
and can include in the page accordingly.
<script src="https://code.jquery.com/jquery-3.5.1.min.js"></script>
- HTML Form is created with Name, Email, Phone Number, Subject and Message textfields and a SEND MESSAGE button.
<form method="POST" action="" id="contact_form">
<label>Enter Name: </label>
<input type="text" name="name" class="form-control" id="name">
<label>Enter Email ID: </label>
<input type="email" name="email" class="form-control" id="email">
<label>Enter Phone: </label>
<input type="text" name="phone" class="form-control" id="phone">
<label>Subject: </label>
<input type="text" name="subject" class="form-control" id="subject">
<label>Message: </label>
<textarea class="form-control" cols="15" rows="5" name="message" id="message">
</textarea>
<input type="hidden" name="action" value="submit_contact__form">
<button type="button" name="message_btn" id="message_btn">SEND MESSAGE</button>
</form>
- Form is posted using jQuery AJAX method and All the jQuery functions are put inside the document ready function to make sure that all the events are executed after the DOM gets ready for it.
$(document).ready(function(){
…
});
- In order to post the form, click event is defined on send message button where you can perform all the required validations and then the form values are posted to
process.php
file. Code is:
$("#message_btn").click(function(){
var name = $("#name").val();
var email = $("#email").val();
var phone = $("#phone").val();
var subject = $("#subject").val();
var message = $("#message").val();
if(name == "" || email == "" || phone == "" || subject == "" || message == ""){
alert("All fields are required.");
}else{
// AJAX METHOD HERE…
}
});
- jQuery Ajax method defines the following parameters.
url: |
url parameter specifies the url you want to request. |
type: |
HTTP GET and POST are used to request the data from the server. |
data: |
It specifies the data to send with the request. |
success: |
Success is invoked upon successful completion of Ajax request. |
error: |
Error is invoked upon the failure of Ajax request. |
$.ajax({
url: 'process.php',
type: 'POST',
data: $("#contact_form").serialize(),
success: function(data){
var arr = JSON.parse(data);
alert(arr['msg']);
$("#contact_form")[0].reset()
}
});
- We have used HTTP POST REQUEST method to post the values to
process.php
file. Data is sent using serialize() method of JQuery. - As response that we have received from php file is JSON encoded, therefore, we have decoded the same using
JSON.parse
jQuery function. - We have also emptied the whole form using reset method.
process.php⬇
<?php
include_once 'includes/conn.php';
if(isset($_POST['action']) && $_POST['action'] == "submit_contact__form"){
$arr['error'] = 0;
$arr['msg'] = "";
$name = $_POST['name'];
$email = $_POST['email'];
$email = filter_var($email, FILTER_SANITIZE_EMAIL);
$email = filter_var($email, FILTER_VALIDATE_EMAIL);
$phone = $_POST['phone'];
$user_subject = $_POST['subject'];
$user_message = $_POST['message'];
if($name != "" && $email != "" && $user_message != ""){
$subject = "Contact US";
$to = "[email protected]";
$from = $email;
$headers = 'MIME-Version: 1.0' . "\r\n";
$headers .= 'Content-type: text/html; charset=iso-8859-1' . "\r\n";
$headers .= 'From: '.$from."\r\n".
'Reply-To: '.$from."\r\n" .
'X-Mailer: PHP/' . phpversion();
$message = '<h3>Contact Form Details</h3>
<table border="1" cellpadding="10">
<tr>
<th>Name</th>
<td>'.$name.'</td>
</tr>
<tr>
<th>Email</th>
<td>'.$email.'</td>
</tr>
<tr>
<th>Phone</th>
<td>'.$phone.'</td>
</tr>
<tr>
<th>Subject</th>
<td>'.$subject.'</td>
</tr>
<tr>
<th>Message</th>
<td>'.$user_message.'</td>
</tr>
</table>';
if(mail($to, $subject, $message, $headers)){
$sql = "INSERT INTO
contact_us(id, name, email, phone, subject, message, status, created_at)
VALUES('', '".$name."', '".$email."', '".$phone."', '".$user_subject."', '".$user_message."', '1', '".date('Y-m-d H:i:s')."')";
$res = mysqli_query($con, $sql);
$row = mysqli_insert_id($con);
if($row > 0){
$arr['msg'] = "Your Details are sucessfully emailed. We will contact you soon.";
}else{
$arr['error'] = 1;
$arr['msg'] = "Error! Please try again later.";
}
}else{
$arr['error'] = 1;
$arr['msg'] = "Email is not sent. Please try again later.";
}
}else{
$arr['error'] = 1;
$arr['msg'] = "Please enter the fields properly.";
}
echo json_encode($arr);
}
?>
- Connection file conn.php is included using PHP file include method. It connects to the “db” database.
include "includes/conn.php";
- Below we have mentioned the code for
Conn.php
file:
<?php
$con = new mysqli("localhost","root","","mydb");
if ($con->connect_errno) {
echo "Failed to connect to MySQL: ".$con->connect_error;
exit();
}
?>
If there is any error in connecting to the database, error message is displayed on the screen and exit method is called to stop further execution of the php code. Otherwise, connection is successfully built and further execution is proceeded.
- If POST variable “action” is set and its value is submit_contact__form then the control comes inside the if block.
if(isset($_POST['action']) && $_POST['action'] == "submit_contact__form"){
}
- Here, we have set
$arr[‘error’]
variable to 0 and$arr[‘msg’]
to “”. Other variables are filtered, validated and are then stored in local variables. - If name, email and message are not empty then control comes inside the if block, otherwise an error is generated.
if($name != "" && $email != "" && $user_message != ""){
// Email and SQL Code goes here.
}else{
$arr['error'] = 1;
$arr['msg'] = "Please enter the fields properly.";
}
- We have mailed all the user details to [email protected] email ID using PHP mail function as:
mail($to, $subject, $message, $headers
where,
to : carries the address to whom the email is being sent.
subject: carries the subject of an email ID.
message: main body content is stored in message variable. HTML tags can also be used here.
headers: all the extra information like “FROM”, “CC”, “BCC” and other additional headers.
- On successful mail sending, the information is stored in contact_us table using mysqli insert statement. If insertion is successful, success message otherwise an error message is generated and is displayed on screen.
echo json_encode($arr);
A PHP array is encoded to JSON array and is echoed. This array is further decoded in JS method as we have discussed it earlier in INDEX.PHP
file.
Summary
In this project, we have learned to mail the HTML form data filled by a user on our website. We have used jQuery AJAX method to post the form values for processing. Values are posted to process.php file where they are validated and then are emailed to specific user ID. If mail is sent, the values are also inserted in a table to keep record of the emails in a table as well. Success and Error messages are also displayed accordingly.