Login and Logout functionality with Session Handling
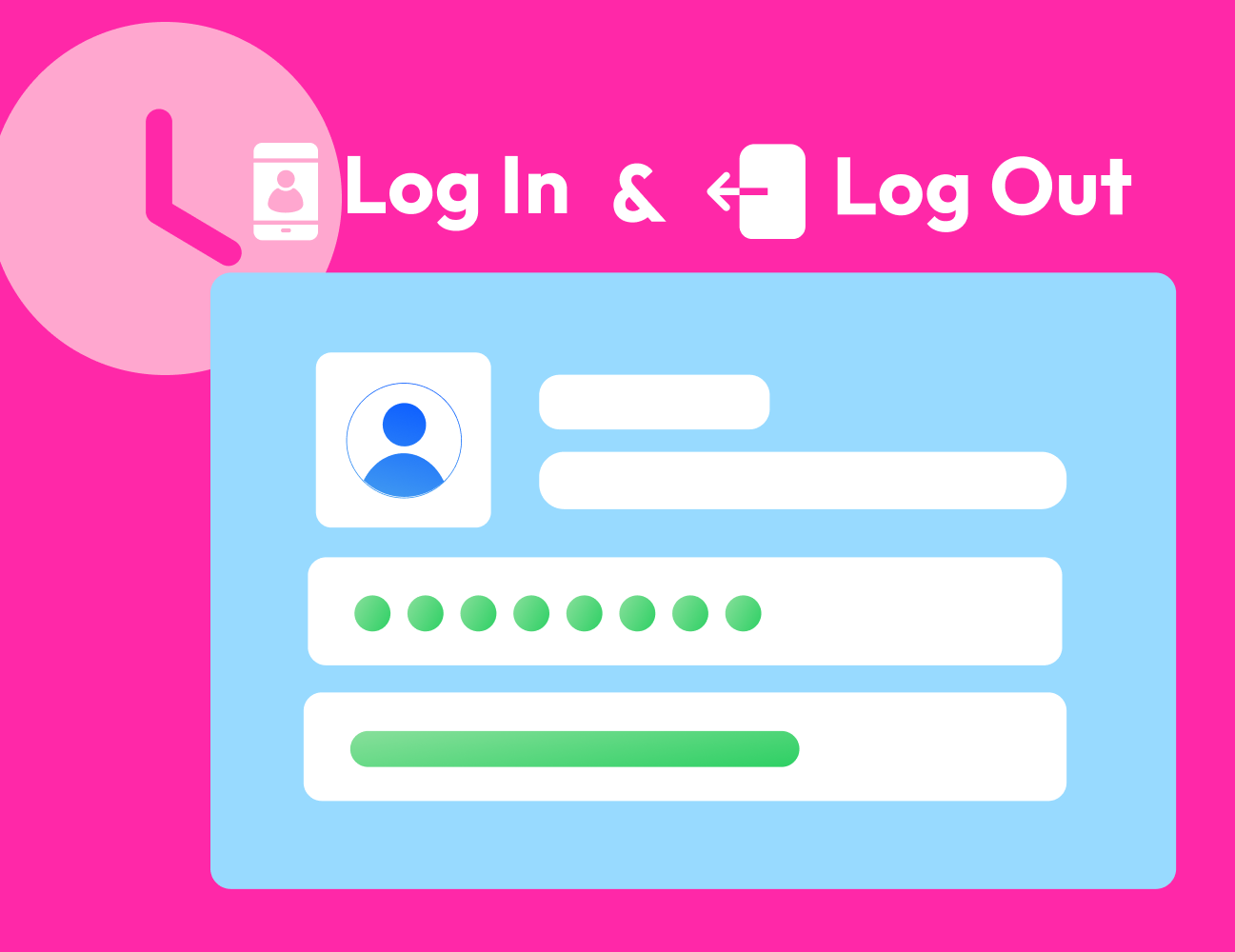
Prerequisite: Basic Understanding of HTML, CSS, PHP and MySQL.
In this project:
- We will create a simple HTML Login Form.
- Username and Password will be taken as input from the user and there will be LOGIN button for submission.
- A users table is created at the backend which stores all the information regarding username, password and status of the users of our website.
- Form is processed and entered credentials will be matched from the table. If user is authenticated, the control will be transferred to the welcome page. Otherwise, error message is displayed on the login page.
- Logout button is fixed on all the session pages. So, a user can logout his ID whenever he wants to.
- Logout just destroys all the sessions and the user is moved to the Login Page again.
index.php ⬇
<?php
@session_start();
if(isset($_SESSION['user_id']) && is_numeric($_SESSION['user_id'])){
header("Location:welcome.php");
}
?>
<!DOCTYPE html>
<html>
<head>
<title>LOGIN</title>
<link rel="stylesheet" href="https://maxcdn.bootstrapcdn.com/bootstrap/3.3.7/css/bootstrap.min.css">
<script src="https://code.jquery.com/jquery-3.5.1.min.js"></script>
<style>
.row{margin-top: 10px}
.login_container h4{text-align: center}
.login_container{background-color: #eeeeee; box-shadow: 10px 10px 10px #ccc; padding: 20px; width: 400px; margin-top: 10%}
.error{color: red}
</style>
</head>
<body>
<div class="container login_container">
<div class="row">
<div class="col-md-12">
<?php
if(isset($_SESSION['msg']) && $_SESSION['msg'] != ''){
echo "<p class='error'>".$_SESSION['msg']."</p>";
unset($_SESSION['msg']);
}
?>
</div>
</div>
<form method="POST" action="process.php">
<div class="row">
<div class="col-md-12">
<input type="text" class="form-control" id="email" name="email" placeholder="Enter username" />
</div>
</div>
<div class="row">
<div class="col-md-12">
<input type="password" class="form-control" id="password" name="password" placeholder="Enter password" />
</div>
</div>
<div class="row">
<div class="col-md-12" style="text-align:center">
<button type="submit" class="btn btn-primary">LOGIN</button>
</div>
</div>
</form>
</div>
</body>
</html>
Explanation of Index.php
- HTML Login Form is created with two input fields of username and password and one LOGIN Button.
<form method="POST" action="process.php">
<input type="text" class="form-control" id="email" name="email" placeholder="Enter username" />
<input type="password" class="form-control" id="password" name="password" placeholder="Enter password" />
<button type="submit" class="btn btn-primary">LOGIN</button>
</form>
- When user clicks on the LOGIN button, variables are posted to the
process.php
for further processing.
<form method="POST" action="process.php">
- If session is already set, LOGIN page is not allowed to open and following instructions are coded for that.
<?php
@session_start();
if(isset($_SESSION['user_id']) && is_numeric($_SESSION['user_id'])){
header("Location:welcome.php");
}
?>
In order to use, PHP Super Global Variable $_SESSION
on any page, we need to first start the session using session_start() method. If session is set, user is moved to the Welcome Page.
- “Users” table is created inside “mydb” database and we have also inserted some dummy rows for login. It may look like:
Where, user_id
is the primary key of the table and is unique to every user.
Status : 1 refers to active users, 0 refers to the inactive users.
- In case of any error message generated, which is supposed to be displayed on Login Page, the following error code is specified:
<?php
if(isset($_SESSION['msg']) && $_SESSION['msg'] != ''){
echo "<p class='error'>".$_SESSION['msg']."</p>";
unset($_SESSION['msg']);
}
?>
- If
$_SESSION[‘msg’]
is set and is not void, the message is displayed on the screen and then we have unset the$_SESSION[‘msg’]
variable so that, it should not display the same error message again and again on reloading.
process.php⬇
<?php
include_once 'includes/conn.php';
@session_start();
$email = $_POST['email'];
$email = filter_var($email, FILTER_SANITIZE_EMAIL);
$email = filter_var($email, FILTER_VALIDATE_EMAIL);
$password = $_POST['password'];
$sql = "SELECT * FROM users WHERE email = '".$email."' AND password = '".$password."' and STATUS = '1'";
$exe = mysqli_query($con, $sql);
$num_row = mysqli_num_rows($exe);
if($num_row > 0){
$row = mysqli_fetch_assoc($exe);
$_SESSION['user_id'] = $row['user_id'];
$_SESSION['username'] = $row['name'];
$_SESSION['msg'] = '';
header("Location:welcome.php");
}else{
$_SESSION['msg'] = "Invalid Username or Password";
header("Location:index.php");
}
?>
Explanation of process.php
- Connection file is included in
process.php
file which creates a connection with mydb database.
<?php
$con = new mysqli("localhost","root","","mydb");
if ($con->connect_errno) {
echo "Failed to connect to MySQL: ".$con->connect_error;
exit();
}
?>
- Form values are posted here which are further validated and stored in local variables
$email
and$password
.
$email = $_POST['email'];
$email = filter_var($email, FILTER_SANITIZE_EMAIL);
$email = filter_var($email, FILTER_VALIDATE_EMAIL);
$password = $_POST['password'];
- User record is fetched from the users table by using WHERE clause in MySQL Query as:
$sql = "SELECT * FROM users WHERE email = '".$email."' AND password = '".$password."' and STATUS = '1'";
If user record is found, SESSION variables are set and user is moved to the Welcome Page. Otherwise, error message is set and the user is moved to Login Page.
welcome.php⬇
<?php
@session_start();
if(!($_SESSION['user_id'])){
$_SESSION['msg'] = "Please login your details.";
header("Location:index.php");
}
?>
<!DOCTYPE html>
<html>
<head>
<title>LOGIN</title>
<link rel="stylesheet" href="https://maxcdn.bootstrapcdn.com/bootstrap/3.3.7/css/bootstrap.min.css">
<script src="https://code.jquery.com/jquery-3.5.1.min.js"></script>
</head>
<body>
<div class="container">
<div class="row">
<div class="col-md-8">
<h3>Hello, <?=$_SESSION['username']?></h3>
<h4>Welcome to our website.</h4>
</div>
<div class="col-md-4" style="padding-top: 20px">
<a href="logout.php">LOGOUT</a>
</div>
</div>
</div>
</body>
</html>
Explanation of welcome.php
- If session is not set, User is moved to Login Page. This instruction is set on all the pages which are User Specific. Code may look like.
if(!($_SESSION['user_id'])){
$_SESSION['msg'] = "Please login your details.";
header("Location:index.php");
}
- Welcome page is coded according to the requirement of our website. We have simply displayed a welcome message to our User alongwith user’s name. A LOGOUT button is also specified on this page.
logout.php⬇
<?php
session_start();
session_destroy();
header("Location:index.php");
?>
Explanation of logout.php
session_destroy()
method is used to empty all the variables in a session array. But if you want to destroy a single session variable then unset() method is used.Header()
in-built PHP method is used to redirect the user to Login Page.
Summary
In this project, we have learned to create a Login page for our website. It is composed of a HTML Form with two input fields i.e. Username and Password and a LOGIN Button. If users enters the correct details, the user is moved to the welcome page, where he can access his profile and other key features of the website as per the requirement of a website. But in case if user enters incorrect username or password, Error message is displayed on screen and user is asked to fill the correct information.
User’s information is stored in session variables which are then accessed through multiple pages. Hence, controlling the access of pages and the information displayed on pages. For instance, no user can open welcome page without entering the login credentials.